Building a Blog: CaaS-Style
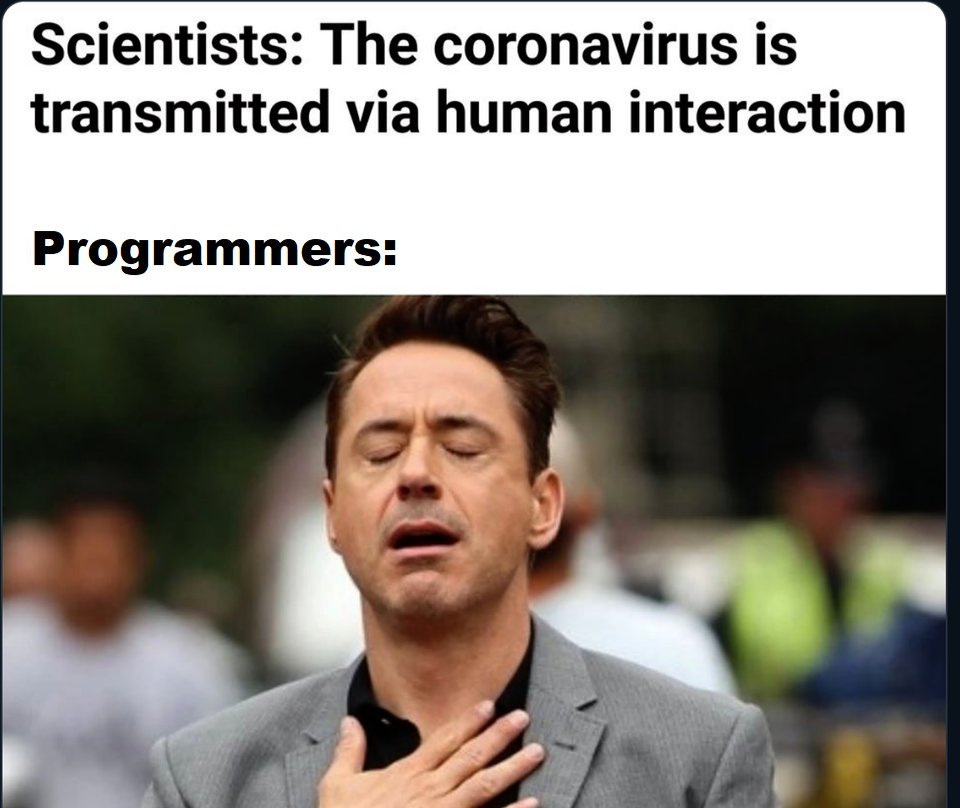
Since starting at Sitecore, and working with Content Hub, I've heard a lot about Experience Edge and Content as a Service (CaaS). The concept, like many aaS's is that by splitting the responsibilities of what would traditionally be one big system, between multiple smaller systems, each can perform their role much better. In this case, for CaaS, we're taking about splitting content creation from content delivery. Marketeers are free to focus on creating great content, without having to interact with the developers (because who wilfully does that?), and developers can take care of delivering beautiful, performant websites, without having to interact with, well anyone (because what dev wilfully interacts with people?). I often learn best through action, so I wanted to try and implement something that made use of CaaS.
Creating the Site
Sitecore Content Hub offers a module called Content Marketing Platform (CMP) which provides an easy way to create various types of content and integrates seamlessly with Sitecore Experience Edge. I decided that I could use this to create a simple personal blog website, it would be a lightweight front end application that could be provided content by Content Hub's CMP module via Experience Edge.
My plan was to develop a static website that I will later hook up to Content Hub to retrieve the blog content. For a number of years my go to front end development stack of choice has been Lit (since it's origin as Polymer), but I figured this was a good opportunity to try something new. I've heard a lot about Next.JS recently, particularly at SUGCON, so I thought I'd give that a go. As luck would have it, the Next.JS tutorial involves creating a simple blog site - perfect! I proceeded through this, and then had a lovely personal blog site, with static generation pulling data from some local markdown files. All I needed to do now, was swap this code out for something that pulled the data from Content Hub.
Configuring Content Hub
The first step of this part is of course to set up an instance of Content Hub. This needs to have both the CMP and Experience Edge modules enabled - I chose to use a Sandbox. The default configuration for blogs that comes with CMP is perfectly sufficient for our needs, so adding these is very simple. I chose to use the two blogs from the Next.JS tutorial.
I then need to check is that the delivery service is configured correctly for our needs. First I checked that the delivery service is enabled, via Manage > Delivery, and then that the specific entities I am after are enabled for delivery - in this case that is M.Content of type Blog. There are some additional things I can configure here, such as which fields are sent to the delivery node, and even under what conditions entities are published, but that's probably best saved for another day.
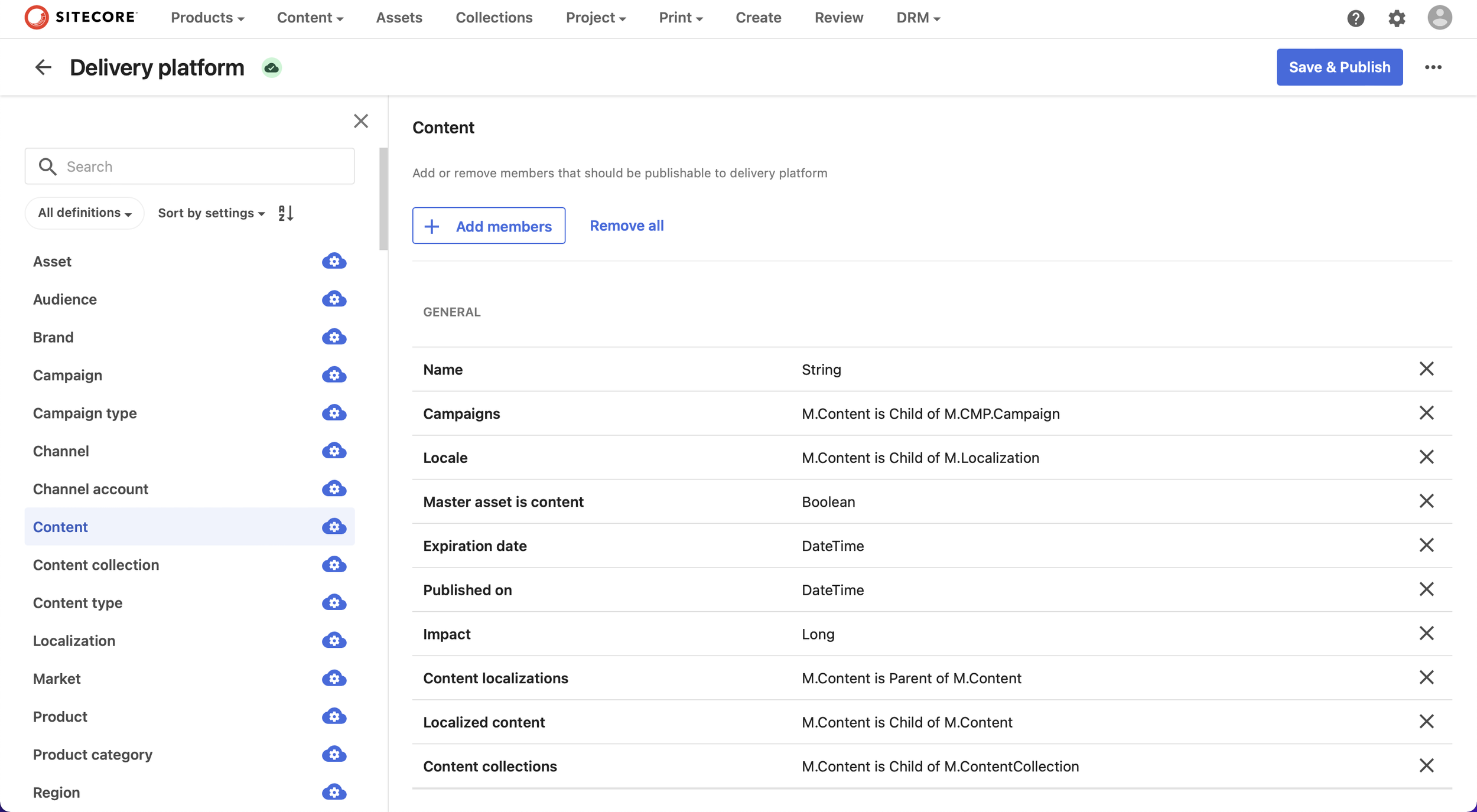
Finally, I need to generate an authentication token for Experience Edge. This is done via Manage > API Keys. For this project I am using the preview service, so I generate a token with the Preview scope and save it for future use.
Making it Work! So now we've got our blog site, and a configured instance of Content Hub, we just need to connect them to each other - easy right? In fact, it is actually really quite easy. I was surprised at how quick this next part came together. Using experience edge, querying data from Content Hub using GQL is as simple as this:
async function getData(query) {
const response = await fetch(`${contentHubBaseUrl}/api/graphql/preview/v1`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
"X-GQL-Token": contentHubGQLToken,
},
body: JSON.stringify({query})
})
const responseObj = await response.json();
return responseObj.data;
}
Where contentHubBaseUrl is the url of your Content Hub base instance, and contentHubGQLToken is the token we generated earlier.
I can then use this to update the post.js file from the Next.JS tutorial to look something like this:
export async function getSortedPostsData() {
const query = "{ blogs:allM_Content_Blog(orderBy:CREATEDON_DESC) { results { id, date:content_PublicationDate, title:blog_Title } } }"
const blogsData = await getData(query);
return blogsData.blogs.results;
}
export async function getAllPostIds() {
const query = "{ blogs:allM_Content_Blog { results { id } } }"
const blogsData = await getData(query);
return blogsData.blogs.results;
}
export async function getPostData(id) {
const query = `{ blog:m_Content_Blog(id:"${id}") { id date:content_PublicationDate title:blog_Title body:blog_Body } }`
const blogData = await getData(query);
return blogData.blog;
}
And that's it! I've now got a blog site that uses static site generation, using content from Content Hub. I can write a blog post in Content Hub, and once I publish it, it is pushed to the delivery service and can be consumed by my site.
I uploaded the repository to GitHub and, after some minor UI changes, connected the repository to Vercel - you can check it out here. I'd love to know what you think and if anyone is doing anything similar with Content Hub and Experience Edge.