Using OAuth with Content Hub Actions
The actions and triggers functionality within Content Hub makes it super easy to integrate with other applications. This combination can be configured to ultimately call and API endpoint when certain conditions are met. If this API endpoint uses OAuth authentication, then some customisation is needed to make this work - let's look at what this entails.
TL;DR Go and check out this repository.
The Problem
Consider the following integration architecture - I know it's simple, we're going to expand it, don't worry.

This setup can easily be achieved using, as mentioned, an API call action from Content Hub. This can also be customised further by setting header and body values. This is useful for adding something like an authorisation header, for example:
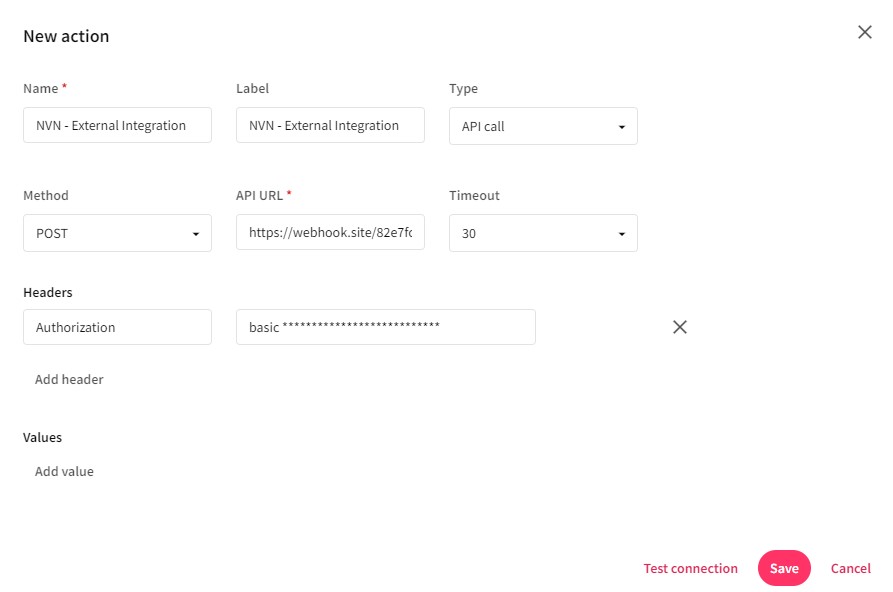
If this authentication header (or any other for that matter) are static, and don't need to change, then that's it - we're done, there's no more to do. However, in some situations, such as when the external service uses OAuth authentication, the values need to change because at some point the token will expire. This is the scenario we're going to focus on here, although the code could be adapted to cover any other scenario where either a payload or header value needs to be periodically updated.
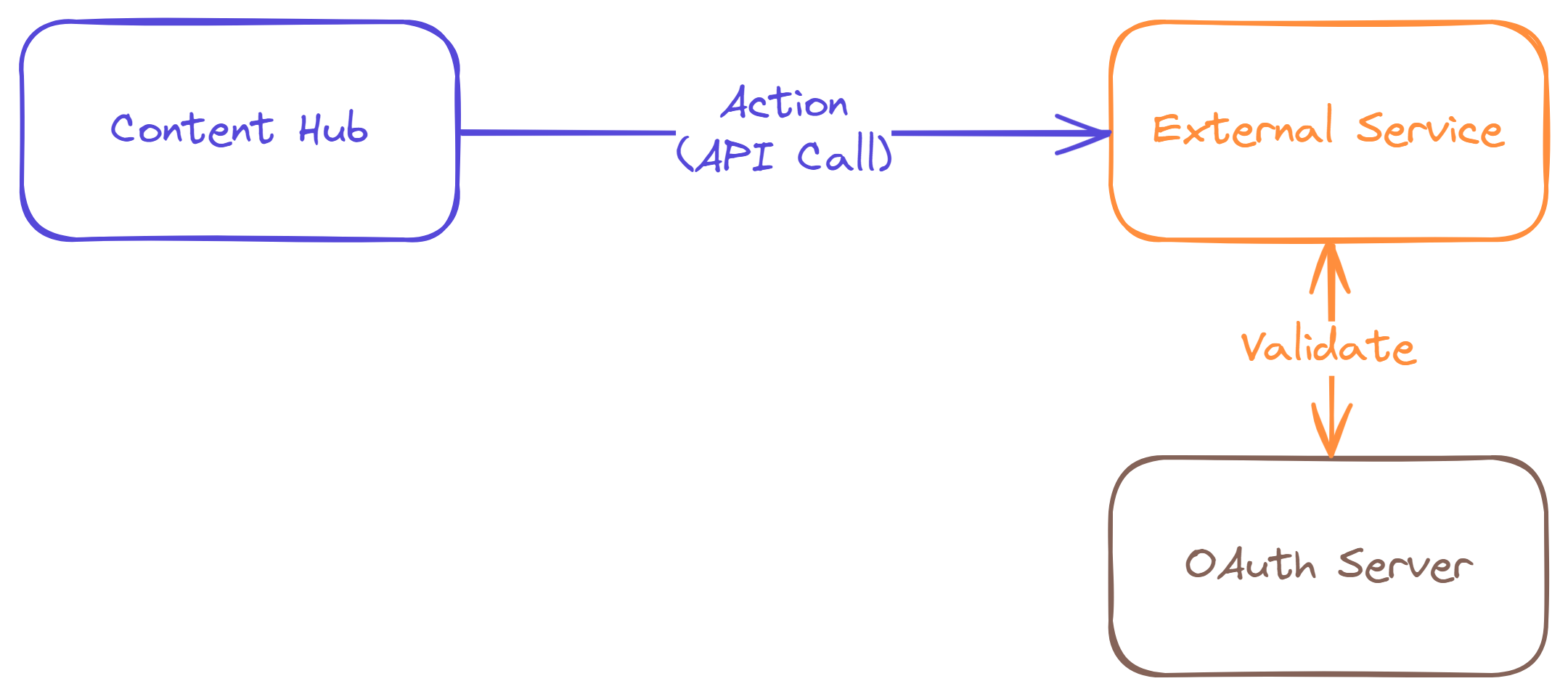
The Solution
Given that Content Hub has no way of scheduling an action to execute periodically, we're going to need something to do this for us. For the example I am going to use an Azure Function with a Timer Trigger.
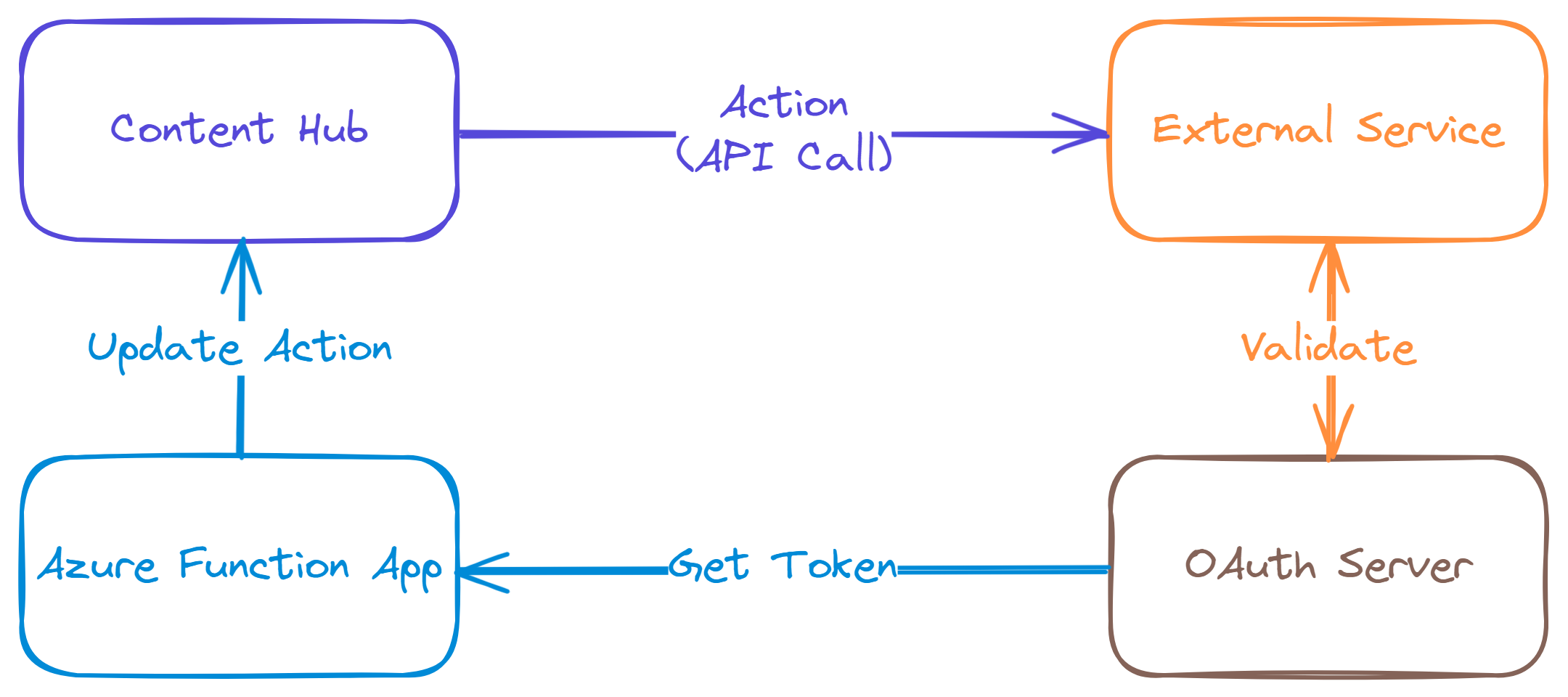
In my case the OAuth server issues tokens with a time of live of 1 hour, so I am going to schedule the function to run every 55 minutes. The function will get a new token from the OAuth server and then update the authorization header of the actions in Content Hub with this new token. Here is a snippet of the code:
[Function("RefreshActionTokens")]
public async Task Run([TimerTrigger("/55 * * * *")] TimerInfo timer)
{
_logger.LogInformation($"Refresh Action Tokens function executed at: {DateTime.Now}");
// Generate a new OAuth token
var authorizationHeader = await _tokenService.GetAuthorizationHeaderAsync();
// Get the Content Hub Actions to update
var actionEntities = await _entityHelper.GetManyAsync(_refreshActionTokensOptions.ActionIdentifiers);
// Update the Authorisation header on each of the actions
_actionHelper.UpdateAuthorizationHeaders(actionEntities, authorizationHeader);
// Save the Actions
await _entityHelper.SaveManyAsync(actionEntities);
_logger.LogInformation($"Tokens updated for actions {string.Join(',', actionEntities.Select(e => e.Identifier))}");
if (timer?.ScheduleStatus is not null)
_logger.LogInformation($"Next timer schedule at: {timer.ScheduleStatus.Next}");
}
I have uploaded the full code to a public repository on GitHub.
#GoForIt
By leveraging Azure Functions and Timer Triggers, you can ensure that your authorisation tokens are always up-to-date, allowing seamless communication between Content Hub and external services that use OAuth. I hope this guide has provided you with the insights and tools needed to implement this solution effectively. Happy coding, and don't forget to check out the full code on GitHub! 🚀